Creating Custom Laravel Blade Directive in Laravel 8
Laravel, one of the most used web frameworks in the world and developed with PHP, comes with Blade template engine. The directives developed for specific purposes such as @section, @yield, @parent, @json
, which come as predefined in Blade, provide great convenience to the user while developing the web application. But sometimes we need to write our own directive for our needs. In this post, I will explain how we can create our own directive in Laravel.
First, we create our directive in the boot()
method in the AppServiceProvider.php class in the Providers folder. As seen in the code block below, we have defined our own directive called my_method.
<?php namespace App\Providers; use Illuminate\Support\ServiceProvider; use Illuminate\Support\Facades\Blade; class AppServiceProvider extends ServiceProvider { /** * Register any application services. * * @return void */ public function register() { // } /** * Bootstrap any application services. */ public function boot() { /**My Custom Blade */ Blade::directive('my_method', function ($value = null) { $value = "| ".$value." |"; return "<h1 style='color: green'> $value </h1>"; }); } }
Usage
We can use the custom directive we created in blade files as follows.
<div class="container"> <div class="row justify-content-md-center"> @my_method('this is my first directive.') </div> </div>
When we run the application file, we will get the following output in the internet browser.
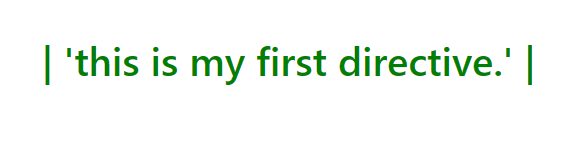
Good luck …