Dynamic Autocomplete Search With typeahead.js
When we design search input field, It is important to show hints or a list of possible choices based on the text that user enter to improve user experices.
In this post, I will show you how to implement this case with html file. If you look through html file below, You can find typeahead.js example. In this example, Some improvements were made.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Typeahed</title> <style> .tt-query, /* UPDATE: newer versions use tt-input instead of tt-query */ .tt-hint, .tt-input { width: 396px; height: 30px; padding: 8px 12px; font-size: 24px; line-height: 30px; border: 2px solid #ccc; border-radius: 8px; outline: none; } .tt-query { /* UPDATE: newer versions use tt-input instead of tt-query */ box-shadow: inset 0 1px 1px rgba(0, 0, 0, 0.075); } .tt-hint { color: #999; } .tt-menu { /* UPDATE: newer versions use tt-menu instead of tt-dropdown-menu */ width: 422px; margin-top: 12px; padding: 8px 0; background-color: #fff; border: 1px solid #ccc; border: 1px solid rgba(0, 0, 0, 0.2); border-radius: 8px; box-shadow: 0 5px 10px rgba(0,0,0,.2); } .tt-suggestion { padding: 3px 20px; font-size: 18px; line-height: 24px; cursor: pointer; } .tt-suggestion:hover { color: #f0f0f0; background-color: #0097cf; } .tt-suggestion p { margin: 0; } .twitter-typeahead{ display: block !important; } </style> </head> <body> <div id="the-basics"> <input class="typeahead border bg-white rounded-pill" type="text" placeholder="Choose State"> </div> <script src="https://code.jquery.com/jquery-2.2.4.min.js"></script> <script src="https://cdn.rawgit.com/julmot/mark.js/6.1.0/dist/jquery.mark.min.js"></script> <script src="https://cdn.rawgit.com/twitter/typeahead.js/v0.11.1/dist/typeahead.bundle.min.js"></script> <script> $(document).ready(function() { var substringMatcher = function(strs) { // console.log('strs', strs); return function findMatches(q, cb) { // console.log('q', q); var matches, substringRegex; // an array that will be populated with substring matches matches = []; console.log('matches', matches); // regex used to determine if a string contains the substring `q` substrRegex = new RegExp('^' + q, 'i'); // iterate through the pool of strings and for any string that // contains the substring `q`, add it to the `matches` array $.each(strs, function(i, str) { if (substrRegex.test(str)) { matches.push(str); } }); // console.log('matches', matches); cb(matches); }; }; var states = ['Alabama', 'Alaska', 'Çevre Mühendisliği', 'Arkansas', 'California', 'Colorado', 'Connecticut', 'Delaware', 'Florida', 'Georgia', 'Hawaii', 'Idaho', 'Illinois', 'Indiana', 'Iowa', 'Kansas', 'Kentucky', 'Louisiana', 'Maine', 'Maryland', 'Massachusetts', 'Michigan', 'Minnesota', 'Mississippi', 'Missouri', 'Montana', 'Nebraska', 'Nevada', 'New Hampshire', 'New Jersey', 'New Mexico', 'New York', 'North Carolina', 'North Dakota', 'Ohio', 'Oklahoma', 'Oregon', 'Pennsylvania', 'Rhode Island', 'South Carolina', 'South Dakota', 'Tennessee', 'Texas', 'Utah', 'Vermont', 'Virginia', 'Washington', 'West Virginia', 'Wisconsin', 'Wyoming' ]; $('#the-basics .typeahead').typeahead({ hint: true, highlight: true, minLength: 1 }, { name: 'states', source: substringMatcher(states) }).bind('typeahead:selected', function (obj, datum) { console.log(obj, datum); }); }); </script> </body> </html>
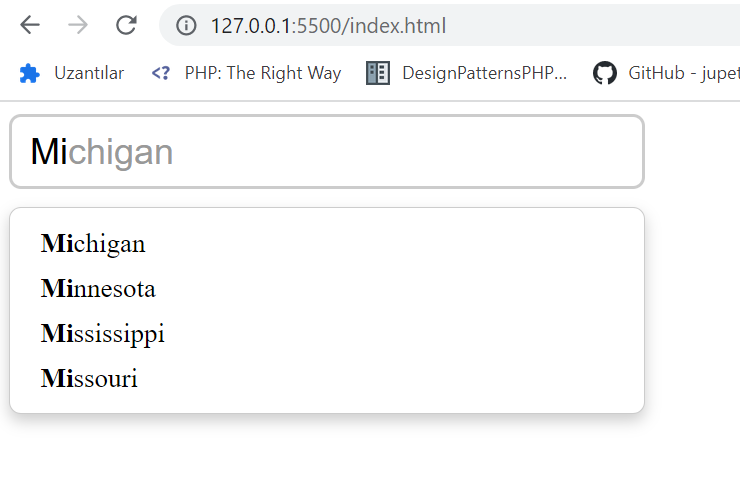
Good Luck …