Writing Unit Test at PHP Laravel
It is important for us to write unit test if we want the codes we developed in a software project to deploy production with the least bugs. Especially if we are going to work in corporate and enterprise companies, we are asked to test the code we have written.
In this post, I will explain how to write a unit test in PHP Laravel and how to run the written test. Writing a test can feel intimidating and a waste of time at first. But as we get used to test writing and practice, we will improve that skill.
Laravel comes with the PHPUnit package where we will run the unit tests. PHPUnit is PHP’s unit testing framework.
First, let’s look at where to write test codes in Laravel. The tests we will write in Laravel are located in the tests folder.
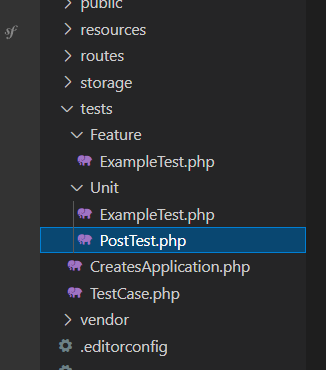
This folder contains Feature and Unit folders and sample tests in these folders.
There are 2 methods of running these tests.
./vendor/bin/phpunit
php artisan test
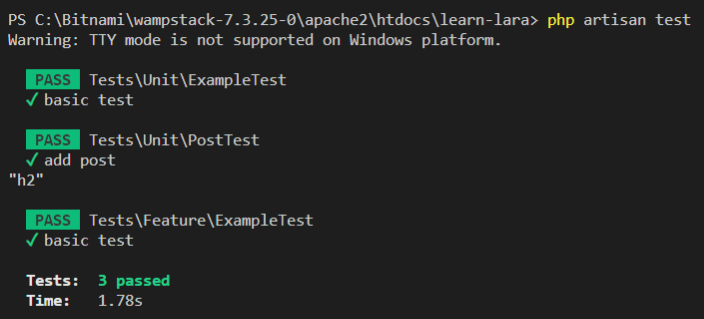
If we only want to run Unit tests, we run php artisan test --testsuite=Unit
command on the terminal screen.
General settings for the tests to be run in Laravel are made in the phpunit.xml file.
Writing Our First Unit Test
In this post, we will write a test for our add_post() method inside our PostsController.
public function add_post(Request $request) { $name = $request->title; $content = $request->content; $status = $request->status; $post = Posts::create([ 'name'=>$name, 'content'=>$content, 'status' =>$status ?? 0 ]); return response()->json($post, 200); }
Kendi testimizi yazmak için ilk önce terminalde php artisan make:test PostTest --unit
komutlarını çalıştırıyoruz. Unit klasörünün altında oluşan PostTest.php dosyasına ilk testimizi yazalım.
To write our own test, we first run php artisan make:test PostTest --unit
commands in the terminal. Let’s write our first test in the PostTest.php file created under the Unit folder.
<?php namespace Tests\Unit; use Tests\TestCase; class PostTest extends TestCase { public function test_add_post() { $response = $this->call('POST', '/admin/add-post', [ 'name' => 'Some post title 123', 'content' => 'Some post content 123', 'status' => false ]); $response->assertStatus($response->status(), 200); } }
To run the test, we run the php artisan test
command in the terminal.
Good luck…