Creating Custom 404 Page in Python Django
When we develop a web application with Django, the user may enter a url that does not exist. In this case, Django will present the user with a standard 404 page that is not stylish. Instead, we can create custom 404 page in Django.
You can learn how to create custom 404 page by following the steps below.
Step 1
In order to see the 404 page we created, it is necessary to change DEBUG status to False in settings.py.
DEBUG = False
Step 2
Next we need to create an alternative 404.html page. We have to create this file in a location we want in the folder where our templates are.
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': [os.path.join(BASE_DIR, 'templates'),], # Templates pathway 'APP_DIRS': True, 'OPTIONS': { 'context_processors': [ 'django.template.context_processors.debug', 'django.template.context_processors.request', 'django.contrib.auth.context_processors.auth', 'django.contrib.messages.context_processors.messages', ], }, }, ]
Step 3
There are predefined handler methods inside the django.urls
functions.
To change this, we add the following line of code to our urls.py file;
from django.urls import path from main.views import * handler404 = 'main.views.handler404' #add to urls.py file app_name = "main" urlpatterns = [ path('', home, name="home"),
We are crushing the existing handler404 function with the handler404 function that will work in this way.
Step 4
We define our handler404 function in the views.py file in our main app.
# 404 PAGE def handler404(request, exception=None): return render(request, 'auth/404.html')
In the picture below, you can see the location of the views.py file in our main app and the file structure of our templates where our 404.html page is located.
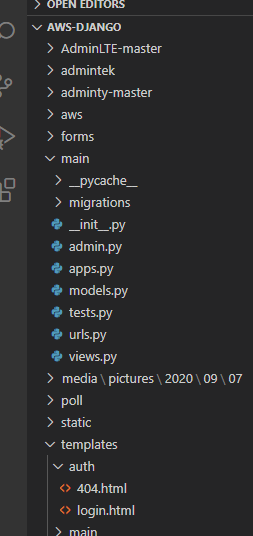
After completing these steps, you can view the 404 page by entering a url that there is not in your application from the browser.