Map, Filter and Reduce Functions in Python
When we write programs in the Python language, we constantly do operations on lists and loops. While doing these operations, python offers us three useful functions that enable us to write our codes in a simple, readable and short way.
In this post, I will explain how to use map, filter and reduce
functions. map and filter functions are built-in functions. However, since the reduce
function is available in the functools module
, we need to import it into our script.
map()
map(function, iterable)
: The map function takes function and iterable as arguments.
Examples:
We want to get 2 times the values on our list as a list. We can do this with both the loop and the map function.
my_list = [1, 2, 3, 4] def two_times(x): return x * 2 #1. Method new_list = [] for i in my_list: new_list.append(two_times ( i )) print(new_list) # Output : [1, 4, 6, 8] #2. Method: with map function output = map(two_times, my_list) print(list(output)) # Output : [1, 4, 6, 8]
Important: the map function returns an object.
If we want to see the result in a list, it is necessary to convert the result to a list with the list() function.
Example: Putting two lists into map function
list_1 = [1, 2, 3, 4, 5] list_2 = [2, 4, 6, 8] def multiply(x, y): return x* y result = map(multiply, list_1, list_2) print(list(result)) # Output : [2, 8, 18, 32]
As you can see, the result list is the size of the smallest list.
Example : Using the map () function with the lambda function
Generally, the map () function is used with the lambda function.
my_list= [1, 2, 3, 4] result = map(lambda x: x**2, my_list) print( list( result ) ) # Output : [1, 4, 9, 16]
The function list can be given as a argument to the map function.
filter()
filter(function, iterable)
: The filter function takes function and iterable as arguments. filter()
forms a new list that contains only elements that satisfy a certain condition.
Example :
my_list = [2, 8, 4, 1, 6, 3, 7, 8 ,9] greater_than_5 = list( filter( lambda x : x >5 , my_list)) print( greater_than_5 ) # Output : [8, 6, 7, 8, 9]
As you can see, the object with values greater than 5 is returned as output, and we converted it into a list with the list () function.
reduce()
reduce(function, iterable)
: The reduce function takes function and iterable as arguments.
from functools import reduce numbers = [1, 3, 5, 7, 9] sum_of_numbers = reduce (lambda x, y : x +y, numbers ) print( sum_of_numbers ) # Output : 49
You can understand how the reduce()
function works from the image below.
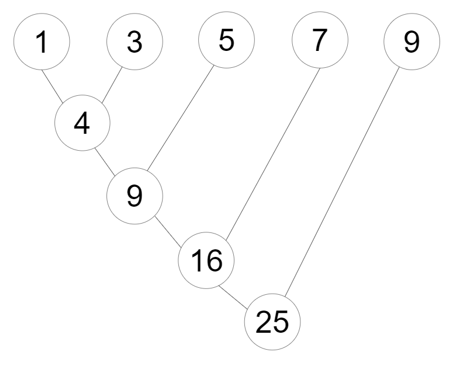
Since the map () and filter () functions are built-in functions of Python, they are a bit faster than for loop.
Finally, these functions should not use too much with lambda functions as it will reduce code readability.