Load Testing Web Applications and Web Services with LOCUST
In this post, I will explain LOCUST, a very useful python library. When we have website or web service, we want the best user experience. For this, we want our website and services to have low response times and not to crash.
Locust is a tool with a user interface that we can quickly and easily test our websites or services performance. With Locust, we can test if our site crashes by making requests to many users at the same time. In addition, we can see how the improvements we made in our application (such as the cache mechanism) are compared to the previous ones by testing Locust.
Installation
In order to use Locust, we first need to install it on our computer. For this, we install it using pip package manager by running the following codes.
After installing library with pip3 install locust
, we can check the version by running locust -v
.
Usage
We create our file named locust_test.py where we will write our tests.
We create our test case by writing the following codes in this test file.
import time from locust import HttpUser, task, between class QuickstartUser(HttpUser): wait_time = between(1, 2.5) @task def my_first_task(self): self.client.get("") @task(2) def my_second_task(self): self.client.get("index.php/about/")
In our test, we will measure the performance of our website by making GET requests to the homepage and about page.
wait_time: Random time between 1 and 2.5 seconds is generated and the HTTP request is made according to this time.
@task : When our test script is run, the tasks defined under the task decorator are performed. task decorator takes an optional parameter.
This parameter defines the weight of the tasks. In our example, my_first_task requests one request while my_second_task requests twice.
Since we created our script on the desktop, we first open the command terminal to run this file. Then we run the following commands by typing (or file_path / locust_test.py):
locust -f locust_test.py

When our script runs without any errors, we can open the Locust interface by typing http: // localhost: 8089/ into the web browser.
Then, a form appears on the screen. In this form, we define how many users will be simulated in the test, in how many seconds we want to add users(spawn rate) and which domain to connect to. When we press the Start swarming button after filling the form, our test starts.
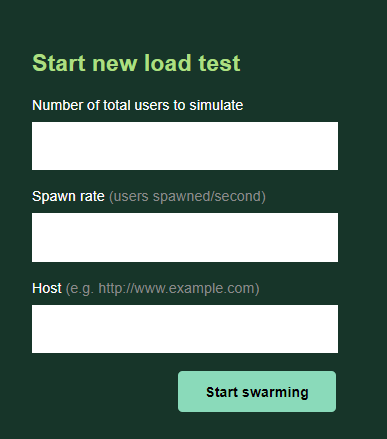
When the test starts, the screen in the picture below will appear. By looking at the table, we can see response times, the total number of requests, the number of requests per second (RPS (request per second)), failures. We can open the chart screen by pressing the Charts tab.
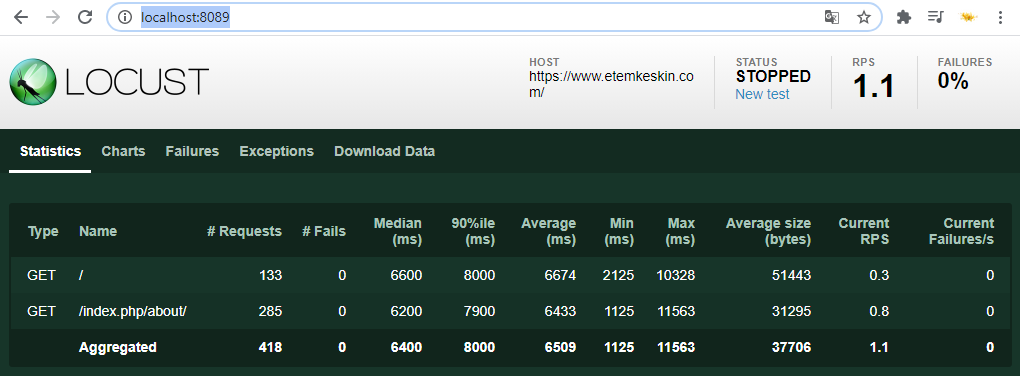
To get the test report, we open the Download Report page and click the download report button to obtain the report.
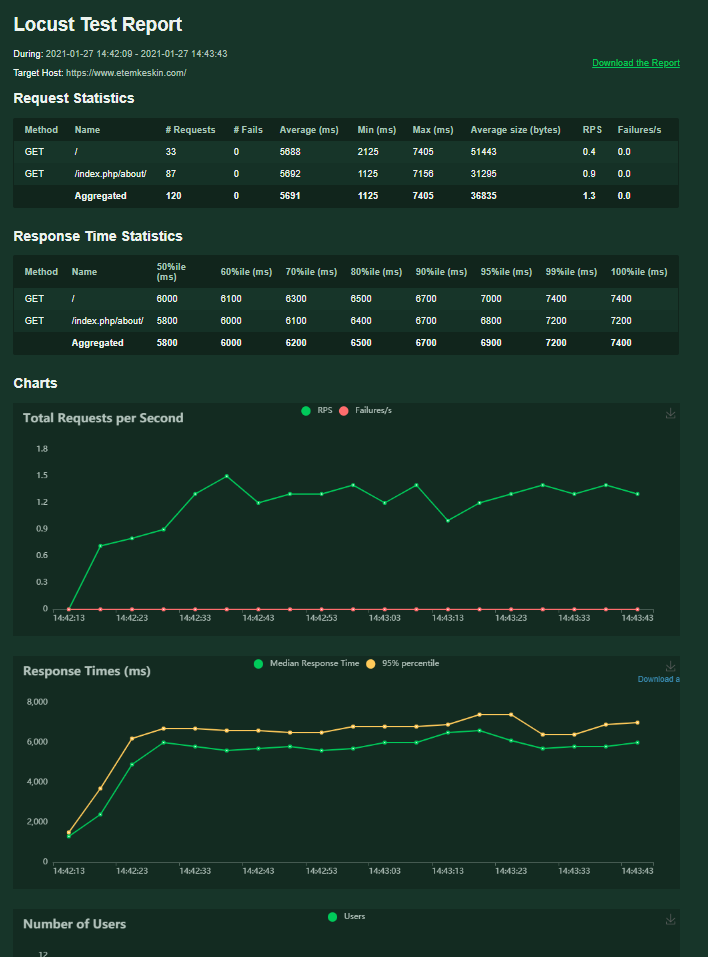
While writing Locust Tests, we can make POST request.
@task def my_load_test(self): self.client.post("/login", json={"username":"foo", "password":"bar"})
We can also define our tests as loop.
@task() def view_items(self): for item_id in range(10): self.client.get(f"/item?id={item_id}", name="/item") time.sleep(1)
Locust also supports using multiple machines for testing. For more information, you can improve your tests using locust’s official document. Good luck.
Sources
- https://docs.locust.io/en/stable/index.html