Printf, print, echo, sprintf, vprintf, vfprintf, vsprintf Statements in PHP
There are many ways to output string expressions in PHP. In this post, I will explain the expressions echo, print, printf, sprintf, vprintf, vfprintf, vsprintf
, which seem to be similar to each other but have various differences, and how they are used.
1.) echo :
- It prints a string output to the screen. String can contain HTML expressions.
- It is not a real function. It comes with the structure of the language.
- It does not return a value when executed. Doesn’t behave like a function
- The echo statement can be used as echo and echo().
- If double quotes are used, variables can be used inside the string expression.
$x = 5; echo "Hello world!"; echo "<h2> PHP is wonderful language. </h2>"; echo "Number is : $x"; // Output: Hello world! // Output: PHP is wonderful language. // Output: Number is : 5
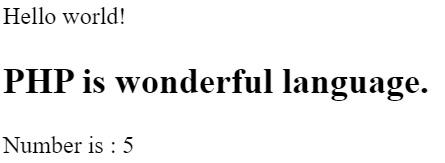
2.) print:
- Outputs a formatted string.
- Since the print statement is not a real function, there is no need to use parentheses. It comes with the structure of the language.
- It always returns 1 when executed.
$x = print "Hello world! <br>"; echo $x; //Output : Hello world! //Output : 1
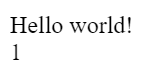
3.) printf:
- Outputs a formatted string.
- Returns the length of the string output when executed.
$number = 5; $str = "London"; $x = printf("There are %u million people in %s. <br>",$number,$str); echo $x; //Output: There are 5 million people in London. //Output: 42

4.) sprintf:
- Returns the formatted string.
$number = 5; $str = "London"; $x = sprintf("There are %u million people in %s. <br>",$number,$str); echo $x; //Output: There are 5 million people in London.

If the arguments are to be used in more than one place, the \$ expression is used.
$number = 5; $str = "London"; $x = sprintf("There are %1\$u million people in %s. <br> Second usage place: %1\$u",$number,$str); echo $x; //Output: There are 5 million people in 5. Second usage place: 5
5.) vprintf:
- Output a formatted string.
- It behaves like printf, except it takes array as an argument instead of variables.
- Returns the length of the formatted string.
$number = 5; $str = "London"; $x = vprintf("There are %u million people in %s. <br>", [$number,$str]); echo $x; //Output: There are 5 million people in London. //Output: 42

6.) vsprintf:
- Returns the formatted string value.
- It behaves like sprintf, except it takes array as an argument instead of variables.
$number = 5; $str = "London"; $x = vsprintf("There are %u million people in %s. <br>", [$number,$str]); echo $x; //Output: There are 5 million people in London.

7.) vfprintf:
- Writes a formatted string to a file.
$number = 5; $str = "London"; $file = fopen("test.txt","w"); vfprintf("There are %u million people in %s. <br>", [$number,$str]);
test.txt dosyasına There are 5 million people in London expression is written in test.txt .
The table below shows the format values you can use for variables in string expressions.
format | POSSIBLE FORMAT VALUES %% – Returns a percent sign %b – Binary number %c – The character according to the ASCII value %d – Signed decimal number (negative, zero or positive) %e – Scientific notation using a lowercase (e.g. 1.2e+2) %E – Scientific notation using a uppercase (e.g. 1.2E+2) %u – Unsigned decimal number (equal to or greather than zero) %f – Floating-point number (local settings aware) %F – Floating-point number (not local settings aware) %g – shorter of %e and %f %G – shorter of %E and %f %o – Octal number%s – String %x – Hexadecimal number (lowercase letters) %X – Hexadecimal number (uppercase letters) |
Good luck…