How to Keep Chrome Browser Open When Using Selenium
With Selenium, we can automate the tests of our web applications. We prepare a test case to test our web application. This test scenario can take several steps.
While conducting our tests, the test may not be able to pass to another without passing one step, as required by the scenario. If our browser is not always open, when we come across a step where our test fails, our test is terminated and it may be necessary to start the test again from the first step. To solve this situation and to use our time more efficiently, we would like to keep our browser open all the time.
In this post, I will explain how we can keep our web browser open for our tests.
We will open our browser in debugging mode in order to keep our Chrome Browser open all the time. For detailed information on how to run Chrome in debugging mode, you can check chrome’s developer documentation at the link below.
https://chromedevtools.github.io/devtools-protocol/
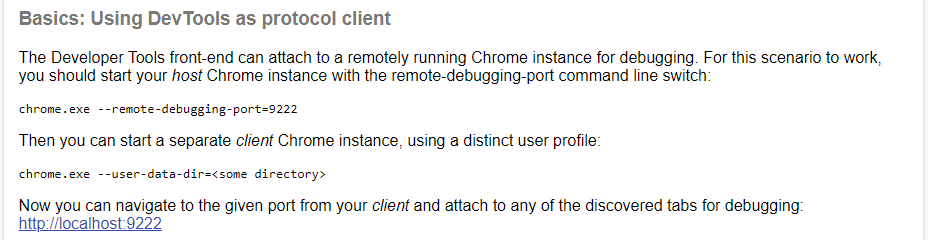
Opening Chrome Browser in Debugging Mode
We create a folder named chromedata where user data will be saved in the folder where chromedriver.exe is located. The name of the file can be any name.

In order to open Chrome Browser in debugging mode, we first open our terminal screen and run the following command to reach chrome.exe file in the folder where chrome is installed. This path may be different on your computer.
cd C:\Program Files\Google\Chrome\Application
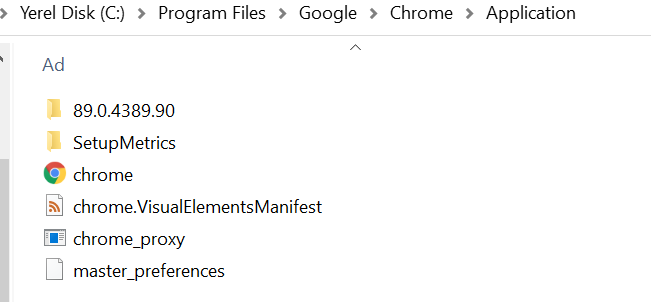
Then we run the commands below.
chrome.exe --remote-debugging-port=9222 chrome.exe --user-data-dir=C:\Users\etem\Desktop\myscript\chromedata
If we run these commands in the terminal screen, the browser opens as follows. We will reach this browser at port 9222.

In the python code block below, I have shown how to use chrome driver.
from selenium import webdriver import time from selenium.webdriver.common.keys import Keys from selenium.webdriver.chrome.options import Options def crawl_website(search_key_send): chrome_options = Options() chrome_options.debugger_address="localhost:9222" browser =webdriver.Chrome(executable_path=r"C:\Users\etem\Desktop\myscript\chromedriver.exe",options=chrome_options) browser.get("https://www.etemkeskin.com/")
Success …